Welcome to another issue!
An update on my new upcoming iOS dev jobs newsletter. It's going to be FREE for my newsletter subscribers. 💪
The link will be sent to you in the next issue. STAY TUNNED! 🚀
I appreciate your support and let's dive into this week's news! 👇

Subscribe to another newsletter I run!
3550 subscribers already! 🚀
Weekly newsletter featuring the best tools for iOS developers. Subscribe if you like @iOS developers
Swift Around the Web
Solve missing API declaration using required reason (ITMS-91053)
You might be getting this error with app submission on the app store. This update requires explaining why your app uses certain user data (like user defaults).
What to do:
- Understand the Error: You'll receive an email with details on missing explanations (e.g., for user defaults).
- Create a Privacy Manifest: (if missing) In Xcode, go to File > New File, search "privacy," and choose App Privacy.
- Find Required Reasons: Use Apple's documentation to find the reason matching your app's data usage.
- Add Reasons to Manifest: In your "PrivacyInfo.xcprivacy" file, list the API category (e.g., user defaults) and the reason (e.g., App Group access).
Deadline: May 1, 2024 (after this, apps without explanations will be rejected).
This guide simplifies the process. Resources and contact info are provided for further assistance.
Swift 6: Access level on import statements
Swift 6 introduces more control over what parts of your dependencies are visible. It changes the default import access level to internal (from public). Explicitly mark public imports to avoid errors.
Previously, sharing code from other sources (like libraries) was like leaving everything out in the open. Now, you can choose what parts to share:
- Secret Tools: Keep hidden helper functions private, like a secret toolbox.
- Public Tools: Share important functions everyone can use, like a public toolkit.
- Less Waiting: Sharing less code might make your project build faster.
This might require some tweaks to existing projects, but overall, it keeps your code cleaner and more organized!
Actor reentrancy in Swift explained
Swift actors are a powerful tool for managing concurrent code with shared state. However, a concept called "actor reentrancy" can introduce unexpected behavior, especially when using asynchronous operations within actors.
Why it matters:
- Imagine an actor that caches data locally and fetches it from a remote server if unavailable and an async read function handles these operations.
- With concurrent read calls, reentrancy can lead to issues like multiple unnecessary network requests are made, wasting resources and slowing down your app. And The actor's state might change during the suspension of the async function (e.g., while waiting for the network call). This can cause unexpected behavior when the function resumes.
- Solution for that: By tracking ongoing tasks within the actor, we can ensure that only one network request happens per data key, even with reentrant calls. This way, the actor reuses existing tasks instead of starting new ones for the same operation.
Understanding actor reentrancy is crucial when using async functions inside actors. By carefully considering state changes and reentrancy implications, you can avoid these pitfalls and write robust concurrent code in Swift.
Safe array subscription in Swift
This post offers a clever solution to prevent app crashes when accessing elements in your Swift arrays.
Using the standard [] subscript on arrays can crash if you try to access an element that doesn't exist.
A custom subscript extension for Collection allows you to access elements safely. It checks the index first and only returns the element if it's valid. Otherwise, it returns nil.
This extension promotes safer and more reliable Swift code by preventing crashes due to out-of-bounds array access.
Coding
Build a chat app using Stream and SwiftUI
This article explores building a basic chat app using Stream and SwiftUI. Here's the gist:
- SwiftUI Package: Integrate Stream's SwiftUI package for pre-built UI components.
- Simple Setup: Connect your app with minimal code using a pre-configured client and dummy credentials (not for production).
- Chat Channel List: Display a list of chat channels using Stream's built-in component.
- Error Handling: Implement basic error handling for login failures.
This is a great starting point to understand how Stream and SwiftUI can simplify chat app development. Remember, proper authentication is crucial for production apps.
Content margins in SwiftUI
SwiftUI's contentMargins modifier brings a powerful tool for managing safe areas in your apps.
Key points:
- The Issue: Scrollable content in SwiftUI, unlike UIKit's readableContentGuide, often leaves unused space on iPads.
- Workaround (Limited): Using safeAreaPadding can shift content, but it also affects toolbars like scrollbars.
- The Solution: contentMargins: This modifier allows fine-grained control over content margins.
By understanding contentMargins, you can create more adaptable and user-friendly interfaces for various screen sizes in SwiftUI.
Design
Creating an App Store Connect-like picker for macOS with SwiftUI
This post dives into creating a custom SwiftUI component for macOS that mimics App Store Connect's beta group picker. It mainly manages beta groups associated with a build: view, add, and remove.
Component Details:
- BetaGroupPicker: Manages interactions with beta groups.
- Binds to the build's beta group list (for edits).
- Takes all available beta groups (for adding options).
- Tracks hovered group (enables removal).
- Displays beta groups and removal buttons.
- Provides a "plus" menu for adding new groups.
Implementation:
- BetaGroup struct represents a group with a name and displayName (showing initials). Updated TestFlightBuildCel integrates BetaGroupPicker.
This custom picker leverages SwiftUI features for a familiar experience, simplifying beta group management in your macOS app.
SwiftUI: Reusable custom style 4 ways
Crafting beautiful and consistent UIs in SwiftUI is all about reusability. This post explores methods to achieve reusable styles in your projects:
- Style Protocols: Perfect for specialized view styles, like buttons with animations. Create a struct following the protocol (e.g., ButtonStyle) and use it with the view's style modifier.
- Custom View Modifiers: Ideal for combining existing modifiers and applying them to different views. Define your style in a struct conforming to ViewModifier and use .modifier(your_style) on any view.
- View Extensions: Similar to modifiers, but avoids repetitive calls. Extend the View protocol with your style function and call it directly on the view (e.g., .neumorphicStyle()).
This empowers you to write cleaner, more maintainable SwiftUI code with a consistent visual language across your app.
Other Cool Stuff
Using your Personal Voice (along with system and novelty voices) in an iOS app
This guide explores how to give your iOS 17 app a voice. Learn how to use built-in voices, let users choose their favorites, and even integrate their own personalized AI voices (with permission!).
The article covers:
- Classic Narration: Easily add voices to your app using system options.
- User Choice: Allow users to pick their preferred voice for a more tailored experience.
- Premium Voices: Offer high-quality downloadable voices for extra pizzazz (downloaded by users).
- Fun & Games (iOS 17): Liven things up with unique voices like cellos or aliens (limited to English-US).
- Personal Touch (iOS 17): Integrate user-created AI voices for a truly personal feel (requires user opt-in).
Get the details on voice selection, permission requests, and compatibility considerations for different devices.
In Case You Missed It
iOS Privacy Manifest - Should developers be worried?
Apple introduces a privacy manifest for iOS apps and SDKs. This informs users how their data is collected (type, reason) and used (linked to user, tracking).
Key Details: Developers disclose data practices through categories, used APIs with justifications, and tracking domains (if applicable). Unclear tracking definitions and lack of specific guidance pose challenges.
Impact on Developers: New requirements may necessitate server-side changes for tracking-related apps. Developers await clarification on webview data, subdomains, and future mandates for non-listed SDKs.
The Future: As the deadline nears, clearer communication and more details are expected to smoothen implementation for developers.
SwiftUI Dynamic Property with KeychainStorage example
This blog post explores creating custom property wrappers in SwiftUI using the DynamicProperty protocol.
Key Benefit: Dynamic properties enable views to update automatically when data changes.
Custom Keychain Storage: We create a KeychainStorage wrapper to securely store and retrieve data from the keychain. It uses @State for internal state management and interacts with a KeychainWrapper for actual keychain access.
Automatic Updates: Whenever the wrappedValue of KeychainStorage changes, the view using it automatically re-renders due to the DynamicProperty conformance.
Example Usage: A LoginCredentials struct is used to demonstrate storing a login timestamp in the keychain. A button press updates the loginCredentials with the current time, triggering a view update and navigation.
Beyond Keychain Storage: The post also briefly touches on building a navigation property wrapper using the coordinator pattern (considered less compatible with SwiftUI).
DynamicProperty empowers developers to create custom data storage solutions that seamlessly integrate with SwiftUI's reactivity system.
Videos
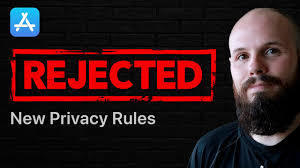
Your app will get rejected | New privacy rules - 2024
Apple's new stricter privacy regulations, effective May 1st, 2024, might be causing your app rejections.
This video tackles this common issue:
- Privacy Manifest Hurdle: You now need a "privacy manifest" for your app and certain third-party libraries (SDKs) you use.
- Most Apps Affected: This likely applies to you because UserDefaults, a common storage method, is considered a "required reason API."
- Easy Fix in Xcode: The video guides you through creating a privacy manifest in Xcode to comply with the new rules.
Watch the video to ensure your app sails smoothly through App Store review.