Welcome to another issue!

Subscribe to another newsletter I run!
Nearly 3700 subscribers already! π
Weekly newsletter featuring the best tools for iOS developers. Subscribe if you like @iOS developers
Sponsored Link

A CI/CD for mobile dev teams trusted by 150,000+ developers
Automate the whole build, test, and release pipeline of your iOS apps to get to the market 20% faster.
Swift Around the Web
Closures vs Async-Await: Asynchronous Operations in iOS
This blog post explores two key approaches for handling asynchronous operations in Swift: closures and async-await.
- Closures: Traditional approach, well-tested, but prone to memory leaks and complex error handling.
- Async-Await (iOS 13+): Modern approach, simplifies code with cleaner syntax and error management, but limited device support.
The best choice depends on your project's specific needs and constraints.
Coding
Xcode 15: Tips & Tricks
List of shortcuts and tricks to boost productivity with Xcode 15 that helps to write code faster and easier. Here's what you'll learn:
- Xcode suggests things: Get help with long lists of options and find the right code pieces quickly.
- Quick access to everything: One shortcut lets you find any feature in Xcode.
- Bookmarks: Mark important lines to easily jump back to them later.
- Smarter context awareness: Xcode suggests symbols, classes, and modifiers based on your code's context.
- Extra: Xcode 15 now fully supports OSLog for efficient app logging.
SwiftUI: Implement Drag to Select for Scrollable LazyVGrid
Create a scrollable grid view in SwiftUI that lets users select multiple items by dragging their finger. This functionality is similar to selecting photos in the iPhone Photos app.
The key is to implement a custom DragToSelectableVGrid view that uses:
- An ItemManager to track item data and selection state.
- A CellView to display each item with a selection indicator.
- Gestures (LongPressGesture and DragGesture) to initiate and handle drag selection.
TipKit for iOS β Exploring the Basics
TipKit empowers iOS developers to guide users towards new or hidden features. This summary dives into the basics:
- TipKit in a Nutshell: A framework for introducing users to app functionalities.
- Benefits: Enhances feature discoverability and user guidance.
- Tip Types: Inline (avoids UI overlap) and Popover (appears above UI).
- Creating Tips: Define content (title, message, image) and display conditions.
- Focus on Clarity: Prioritize actionable instructions for non-obvious features.
visionOS
Understanding typography in visionOS
VisionOS demands special attention to typography. Here's what you need to know:
- Fonts: Use system fonts for optimal readability. Bold and slightly larger fonts are preferred for visionOS compared to iOS.
- Large Titles: Use "Extra Large Title" styles for prominent headings in wide layouts.
- Ditch 3D text: Stick to 2D for clarity from any angle.
- High contrast: White text on dark backgrounds is ideal.
- Vibrancy Helps: Use primary, secondary, or tertiary vibrancy to boost text contrast.
Design
Using materials with SwiftUI
SwiftUI's materials offer a simple solution for improving the clarity of your app's interface. These translucent layers blur the background, ensuring text and other elements remain crisp and easy to read.
Key Benefits:
- Improved readability, especially for complex backgrounds.
- Seamless integration with dark and light mode.
- Material Options: Choose from various material thicknesses for the perfect level of translucency.
- Simple Application: Apply materials with a single modifier for quick implementation.
By leveraging materials, you can create user-friendly and visually appealing interfaces in SwiftUI.
Other Cool Stuff
GeometryReader secret ability for iOS Developer
The post highlights and explores a lesser-known use case for GeometryReader in SwiftUI.
- Beyond Measurements: It can function as a container view, similar to UIKit.
- Key Benefit: Stops the keyboard from pushing up the entire parent view.
- Scenario: A custom sheet view needs to maintain its size despite the keyboard.
- Solution: Apply .edgesIgnoringSafeArea(.bottom) to a GeometryReader.
This approach avoids hidden content issues on smaller devices, unlike traditional solutions.
Swift sucks at web serving⦠or does it?
The post details a debugging process that, although unrelated to iOS development itself, offers valuable takeaways for Swift and iOS developers.
A (faulty) benchmark included Vapor (used for iOS apps). While results are inaccurate, it provides a general reference for iOS web framework performance.
More importantly, the debugging process revealed a way to improve Swift code. This information can help you to identify potential performance bottlenecks in their code to write faster Swift programs.
React to network status updates in SwiftUI
A streamlined approach to monitor network changes in SwiftUI. It leverages the NWPathMonitor to create an async sequence, updating your views efficiently.
The example demonstrates how to:
- Use NWPathMonitor for an async sequence of network status updates.
- Update view state (isNetworkAvailable) based on the connection status.
- The task modifier automatically cancels the sequence when the view disappears.
This method simplifies network monitoring in SwiftUI, promoting clean and efficient code.
In Case You Missed It
Demystifying Variadic Parameters in Swift: A Comprehensive Guide
Swift's variadic parameters let your functions accept a flexible number of inputs. This summary explains what they are and why they're useful.
- What are they? Variadic parameters allow functions to take any number of values of a specific type.
- Why use them? They make functions more adaptable and your code cleaner. Use Cases: Concatenate strings, find maximum values, process dynamic data lists.
By leveraging variadic parameters, you can write cleaner and more adaptable Swift code.
Tutorials
Avoid These Common Errors When Switching from UIKit to SwiftUI
Key mistakes UIKit developers make when switching to SwiftUI:
- Master @Binding & Redraws: Understand two-way data flow and leverage SwiftUI's automatic updates.
- Dynamic & Flexible Layouts: Use .font(.callout), .padding, and .minWidth for accessibility and adaptation.
- Minimize GeometryReader: Favor .overlay() or .background() for simpler layouts.
- ViewModifiers & Built-in Features: Control appearance with ViewModifiers and leverage SwiftUI's functionality.
Following these steps helps write cleaner, more efficient, and adaptable SwiftUI code.
Videos
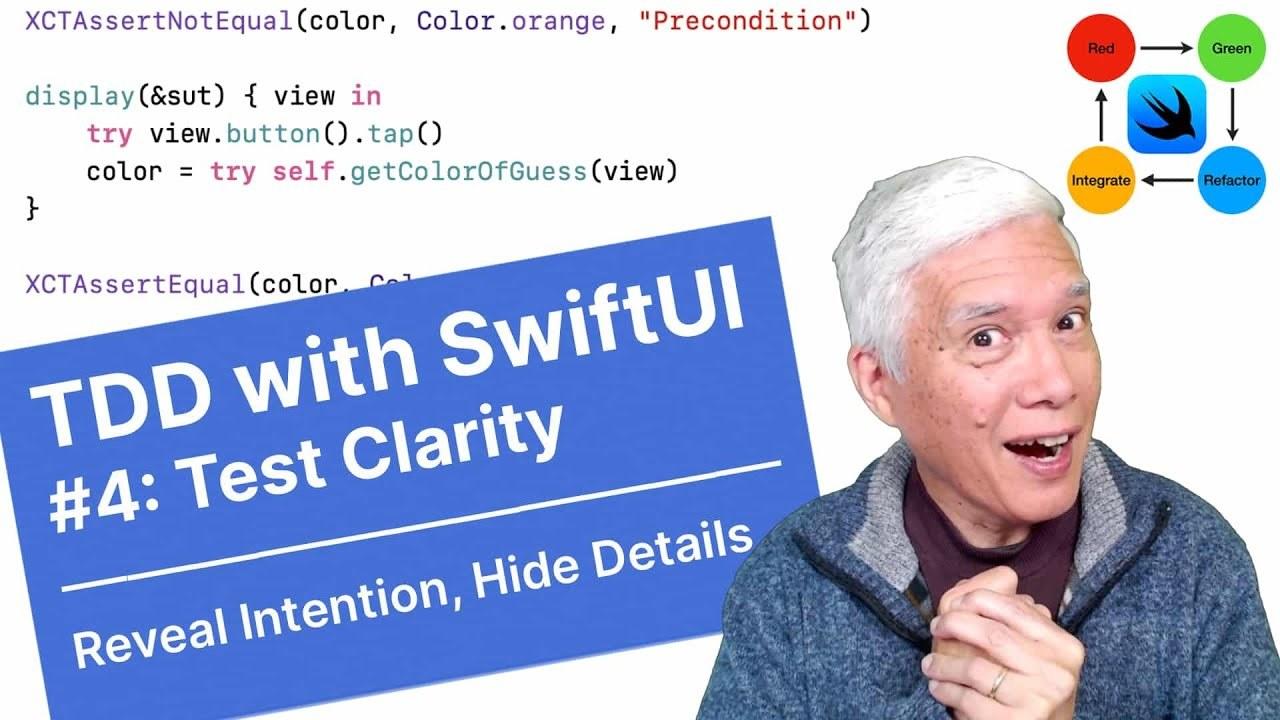
Improve Test Clarity (TDD with SwiftUI)
Learn to write clear and concise unit tests for SwiftUI apps using Test-Driven Development (TDD) with this video.
The key idea is to create tests that focus on the intended outcome, rather than getting bogged down in implementation details.
The video demonstrates how to improve a single SwiftUI microtest, showcasing the benefits of a clear testing approach. This session is part of a live coding series exploring TDD with Swift and SwiftUI.